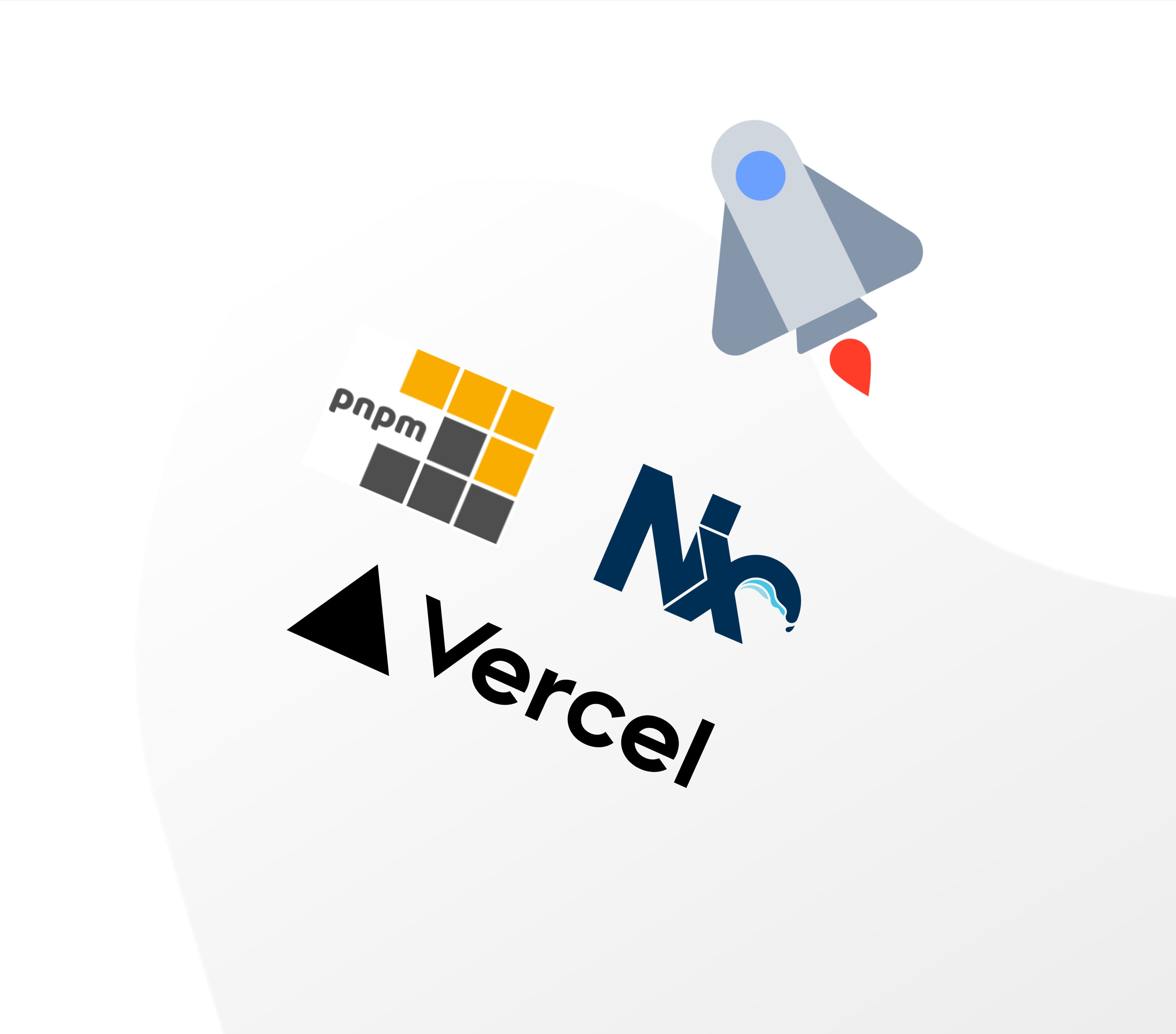
Deploying a multi-application monorepo to the moon
Introduction
In this post, we will explore how to use tools like Vercel, PNPM, and Nx to build and deploy a multi-application monorepo. We'll cover the basics of each tool, the challenges of deploying single vs. multiple applications, and how to set up an effective deployment pipeline that works well with monorepos.
👉 Vercel
Vercel is a cloud platform that helps developers deploy and scale modern web projects. It supports front-end frameworks like Next.js, React, Vue, and others. By providing CI/CD pipelines and serverless functions, Vercel makes it simple to deploy applications.
👉 PNPM
PNPM (Performant NPM) is a fast, space-efficient package manager. When managing dependencies, PNPM stores them in a way that avoids duplications and reduces the overall storage space required. This is a very useful feature for monorepos with multiple projects, as it reduces the installation times significantly.
👉 Nx
Nx is a popular build system that supports monorepos out-of-the-box. It provides powerful tools to manage, build, and test large codebases with multiple applications and libraries. Nx helps build development workflows, enforces best practices, and optimizes builds by having a clear overview of the project's dependencies.
Deploying an application
A visualization of a simple deployment process in a single application
If you are working on a simple application, deploying it is pretty straightforward. The CI/CD pipeline focuses on one codebase, one set of dependencies, and one deployment target.
A visualization of a complex deployment process in a monorepo
A monorepo is composed of multiple applications and libraries within a single repository. Each application can be developed and deployed independently.
Many developers prefer monorepos because they have several advantages, including shared dependencies, consistent tooling, and easier code sharing. On the other hand, working on a large monorepo introduces challenges:
-
Dependency management — ensuring all projects use compatible versions of shared dependencies.
-
Build coordination — building and testing only the affected parts of the repository.
-
Deployment complexity — managing deployment configurations and environments for multiple applications.
Luckily, combining Vercel, PNPM, and Nx might help with solving all three!
How to deploy in Vercel?
A visualization of a simple deployment trigger in Vercel
Vercel can be set up to trigger deployments on specific events, such as merges to the master branch. To do that, you might need to configure a couple of things first:
👉 Enable automatic deployments
In the Vercel dashboard, you can enable automatic deployments for branches. Vercel will automatically deploy changes pushed to the configured branches.
👉 Configure conditional task execution
You can use the Nx's affected
commands to optimize deployments. Nx can determine which projects are affected by changes and Vercel can be configured to deploy only those.
{
"build": {
"script": "nx affected:build"
},
"deploy": {
"script": "vercel --prod"
}
}
👉 Integrate with Github Actions
To make sure that conditional deployments are properly configured, you can add a GitHub Actions workflow to integrate Nx and Vercel:
name: Deploy
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout repository
uses: actions/checkout@v2
- name: Install dependencies
run: pnpm install
- name: Build affected projects
run: npx nx affected:build --base=origin/main --head=HEAD
- name: Deploy to Vercel
run: vercel --prod
env:
VERCEL_TOKEN: ${{ secrets.VERCEL_TOKEN }}
👉 Configure routing
A visualization of deploying multiple applications within a monorepo to multiple sub-domains
Vercel supports deploying multiple applications from a monorepo using its built-in configuration. Each application can be assigned a unique sub-domain. You can specify the domains by adding the configuration to the vercel.json
file at the root of your monorepo:
{
"projects": {
"app1": {
"src": "apps/app1",
"alias": "app1.yourdomain.com"
},
"app2": {
"src": "apps/app2",
"alias": "app2.yourdomain.com"
},
"app3": {
"src": "apps/app3",
"alias": "app3.yourdomain.com"
}
}
}
In the Vercel dashboard, you can configure custom domains and link them to the respective sub-domains defined in vercel.json
.
A screenshot from the Vercel application that shows the domain configuration page
Conclusion
Deploying applications might be complicated, especially when working with large monorepos. Luckily, by combining tools like Vercel with Nx and PNPM, you can prepare a setup for deploying monorepos containing multiple applications to individual targets, all while handling conditional task execution and deployment triggers.